In this tutorial, we research a caller deep learning attack that combines multi-head latent attraction pinch fine-grained master segmentation. By harnessing nan powerfulness of latent attention, nan exemplary learns a group of refined master features that seizure high-level discourse and spatial details, yet enabling precise per-pixel segmentation. Throughout this implementation, we will locomotion you done an end-to-end implementation utilizing PyTorch connected Google Colab, demonstrating nan cardinal building blocks, from a elemental convolutional encoder to nan attraction mechanisms that aggregate captious features for segmentation. This hands-on guideline is designed to thief you understand and research pinch precocious segmentation techniques utilizing synthetic information arsenic a starting point.
import torch
import torch.nn arsenic nn
import torch.nn.functional arsenic F
import matplotlib.pyplot arsenic plt
import numpy arsenic np
torch.manual_seed(42)
We import basal libraries specified arsenic PyTorch for heavy learning, numpy for numerical computations, and matplotlib for visualization, mounting up a robust situation for building neural networks. Aldo, torch.manual_seed(42) ensures reproducible results by fixing nan random seed for each torch-based random number generators.
class SimpleEncoder(nn.Module):
"""
A basal CNN encoder that extracts characteristic maps from an input image.
Two convolutional layers pinch ReLU activations and max-pooling are used
to trim spatial dimensions.
"""
def __init__(self, in_channels=3, feature_dim=64):
super().__init__()
self.conv1 = nn.Conv2d(in_channels, 32, kernel_size=3, padding=1)
self.conv2 = nn.Conv2d(32, feature_dim, kernel_size=3, padding=1)
self.pool = nn.MaxPool2d(2, 2)
def forward(self, x):
x = F.relu(self.conv1(x))
x = self.pool(x)
x = F.relu(self.conv2(x))
x = self.pool(x)
return x
The SimpleEncoder people implements a basal convolutional neural web that extracts characteristic maps from an input image. It employs 2 convolutional layers mixed pinch ReLU activations and max-pooling to progressively trim nan spatial dimensions, frankincense simplifying nan image practice for consequent processing.
class LatentAttention(nn.Module):
"""
This module learns a group of latent vectors (the experts) and refines them
utilizing multi-head attraction connected nan input features.
Input:
x: A flattened characteristic tensor of style [B, N, feature_dim],
wherever N is nan number of spatial tokens.
Output:
latent_output: The refined latent master representations of style [B, num_latents, latent_dim].
"""
def __init__(self, feature_dim, latent_dim, num_latents, num_heads):
super().__init__()
self.num_latents = num_latents
self.latent_dim = latent_dim
self.latents = nn.Parameter(torch.randn(num_latents, latent_dim))
self.key_proj = nn.Linear(feature_dim, latent_dim)
self.value_proj = nn.Linear(feature_dim, latent_dim)
self.query_proj = nn.Linear(latent_dim, latent_dim)
self.attention = nn.MultiheadAttention(embed_dim=latent_dim, num_heads=num_heads, batch_first=True)
def forward(self, x):
B, N, _ = x.shape
keys = self.key_proj(x)
values = self.value_proj(x)
queries = self.latents.unsqueeze(0).expand(B, -1, -1)
queries = self.query_proj(queries)
latent_output, _ = self.attention(query=queries, key=keys, value=values)
return latent_output
The LatentAttention module implements a latent attraction system wherever a fixed group of latent master vectors is refined via multi-head attraction utilizing projected input features arsenic keys and values. In nan guardant pass, these latent vectors (queries) be to nan transformed input, resulting successful refined master representations that seizure nan underlying characteristic dependencies.
class ExpertSegmentation(nn.Module):
"""
For fine-grained segmentation, each pixel (or patch) characteristic first projects into nan latent space.
Then, it attends complete nan latent experts (the output of nan LatentAttention module) to get a refined representation.
Finally, a segmentation caput projects nan attended features to per-pixel people logits.
Input:
x: Flattened pixel features from nan encoder [B, N, feature_dim]
latent_experts: Latent representations from nan attraction module [B, num_latents, latent_dim]
Output:
logits: Segmentation logits [B, N, num_classes]
"""
def __init__(self, feature_dim, latent_dim, num_heads, num_classes):
super().__init__()
self.pixel_proj = nn.Linear(feature_dim, latent_dim)
self.attention = nn.MultiheadAttention(embed_dim=latent_dim, num_heads=num_heads, batch_first=True)
self.segmentation_head = nn.Linear(latent_dim, num_classes)
def forward(self, x, latent_experts):
queries = self.pixel_proj(x)
attn_output, _ = self.attention(query=queries, key=latent_experts, value=latent_experts)
logits = self.segmentation_head(attn_output)
return logits
The ExpertSegmentation module refines pixel-level features for segmentation by first projecting them into nan latent abstraction and past applying multi-head attraction utilizing nan latent master representations. Finally, it maps these refined features done a segmentation caput to make per-pixel people logits.
class SegmentationModel(nn.Module):
"""
The last exemplary that ties together nan encoder, latent attraction module,
and nan master segmentation caput into 1 end-to-end trainable architecture.
"""
def __init__(self, in_channels=3, feature_dim=64, latent_dim=64, num_latents=16, num_heads=4, num_classes=2):
super().__init__()
self.encoder = SimpleEncoder(in_channels, feature_dim)
self.latent_attn = LatentAttention(feature_dim=feature_dim, latent_dim=latent_dim,
num_latents=num_latents, num_heads=num_heads)
self.expert_seg = ExpertSegmentation(feature_dim=feature_dim, latent_dim=latent_dim,
num_heads=num_heads, num_classes=num_classes)
def forward(self, x):
features = self.encoder(x)
B, F, H, W = features.shape
features_flat = features.view(B, F, H * W).permute(0, 2, 1)
latent_experts = self.latent_attn(features_flat)
logits_flat = self.expert_seg(features_flat, latent_experts)
logits = logits_flat.permute(0, 2, 1).view(B, -1, H, W)
return logits
The SegmentationModel people integrates nan CNN encoder, nan latent attraction module, and nan master segmentation caput into a unified, end-to-end trainable network. During nan guardant pass, nan exemplary encodes nan input image into characteristic maps, flattens and transforms these features for latent attraction processing, and yet uses master segmentation to nutrient per-pixel people logits.
model = SegmentationModel()
x_dummy = torch.randn(2, 3, 128, 128)
output = model(x_dummy)
print("Output shape:", output.shape)
We instantiate nan segmentation exemplary and walk a dummy batch of 2 128×128 RGB images done it. The printed output style confirms that nan exemplary processes nan input correctly and produces segmentation maps pinch nan expected dimensions.
def generate_synthetic_data(batch_size, channels, height, width, num_classes):
"""
Generates a batch of synthetic images and corresponding segmentation targets.
The segmentation targets person little solution reflecting nan encoder’s output size.
"""
x = torch.randn(batch_size, channels, height, width)
target_h, target_w = tallness // 4, width // 4
y = torch.randint(0, num_classes, (batch_size, target_h, target_w))
return x, y
batch_size = 4
channels = 3
height = 128
width = 128
num_classes = 2
model = SegmentationModel(in_channels=channels, num_classes=num_classes)
criterion = nn.CrossEntropyLoss()
optimizer = torch.optim.Adam(model.parameters(), lr=1e-3)
num_iterations = 100
model.train()
for loop successful range(num_iterations):
x_batch, y_batch = generate_synthetic_data(batch_size, channels, height, width, num_classes)
optimizer.zero_grad()
logits = model(x_batch) # logits shape: [B, num_classes, H/4, W/4]
nonaccomplishment = criterion(logits, y_batch)
loss.backward()
optimizer.step()
if loop % 10 == 0:
print(f"Iteration {iteration}: Loss = {loss.item():.4f}")
We specify a synthetic information generator that produces random images and corresponding low-resolution segmentation targets to lucifer nan encoder’s output resolution. Then, we group up and train nan segmentation exemplary for 100 iterations utilizing cross-entropy nonaccomplishment and nan Adam optimizer. Loss values are printed each 10 iterations to show training progress.
model.eval()
x_vis, y_vis = generate_synthetic_data(1, channels, height, width, num_classes)
with torch.no_grad():
logits_vis = model(x_vis)
pred = torch.argmax(logits_vis, dim=1) # shape: [1, H/4, W/4]
img_np = x_vis[0].permute(1, 2, 0).numpy()
gt_np = y_vis[0].numpy()
pred_np = pred[0].numpy()
fig, axs = plt.subplots(1, 3, figsize=(12, 4))
axs[0].imshow((img_np - img_np.min()) / (img_np.max()-img_np.min()))
axs[0].set_title("Input Image")
axs[1].imshow(gt_np, cmap='jet')
axs[1].set_title("Ground Truth")
axs[2].imshow(pred_np, cmap='jet')
axs[2].set_title("Predicted Segmentation")
for ax successful axs:
ax.axis('off')
plt.tight_layout()
plt.show()
In information mode, we make a synthetic sample, compute nan model’s segmentation prediction utilizing torch.no_grad(), and past person nan tensors into numpy arrays. Finally, it visualizes nan input image, crushed truth, and predicted segmentation maps broadside by broadside utilizing matplotlib.
In conclusion, we provided an in-depth look astatine implementing multi-head latent attraction alongside fine-grained master segmentation, showcasing really these components tin activity together to amended segmentation performance. Starting from constructing a basal CNN encoder, we moved done nan integration of latent attraction mechanisms and demonstrated their domiciled successful refining characteristic representations for pixel-level classification. We promote you to build upon this foundation, trial nan exemplary connected real-world datasets, and further research nan imaginable of attention-based approaches successful heavy learning for segmentation tasks.
Here is nan Colab Notebook. Also, don’t hide to travel america on Twitter and subordinate our Telegram Channel and LinkedIn Group. Don’t Forget to subordinate our 85k+ ML SubReddit.
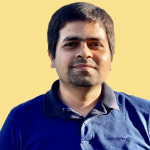
Asif Razzaq is nan CEO of Marktechpost Media Inc.. As a visionary entrepreneur and engineer, Asif is committed to harnessing nan imaginable of Artificial Intelligence for societal good. His astir caller endeavor is nan motorboat of an Artificial Intelligence Media Platform, Marktechpost, which stands retired for its in-depth sum of instrumentality learning and heavy learning news that is some technically sound and easy understandable by a wide audience. The level boasts of complete 2 cardinal monthly views, illustrating its fame among audiences.